Generate and verify signatures
To ensure the security and integrity of data exchanges between apps and SHOPLINE, you need to implement signing and signature verification procedures in specific scenarios.
- Generate a signature for your HTTP requests sent to SHOPLINE.
- Verify SHOPLINE's signature on incoming HTTP requests.
This article guides you on how to use the HMAC-SHA256 algorithm to sign and verify requests, preventing tampering during transmission. For detailed information on the HMAC-SHA256 algorithm, refer to HMAC and SHA-2.
Use cases
App authorization
Before you use Admin Rest APIs and Admin GraphQL APIs, your app needs to complete the app authorization to obtain an access token. In the authorization process, you need to sign requests and verify signatures sent by SHOPLINE. For details about when to generate and verify signatures, refer to App authorization.
Verify signatures for webhooks
When SHOPLINE sends webhooks to you, you need to verify the signature passed together with the webhook. For more information about how to subscribe webhook events, refer to Webhooks.
Operation procedure
Prerequisites
- Check whether the request uses the GET or POST method and proceed accordingly.
- Obtain the app secret for generating and verifying the signature by following these steps:
- Log in to the SHOPLINE Developer Center.
- Go to Apps.
- Click your target app name, and you will find the app secret under App credentials.
If you reset your app secret, ensure to update the app secret used in the algorithm to prevent signature generation or verification failures.
GET requests
Sign a request
- Generate
source
to be signed: Encode the query parameters of the request with URL encoding. Then sort the query parameters in alphabetical order to create thesource
string. - Generate a signature: Generate a signature by encrypting
source
using the HMAC-SHA256 algorithm and the app secret obtained in the prerequisite. - Add the signature to the request: Add the signature
sign
to the URL query parameters to construct the final query parameters of the request.
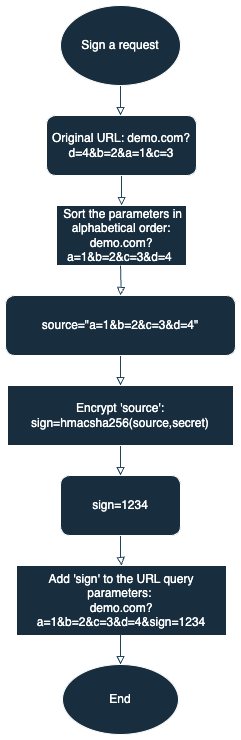
Verify a signature
- Get the signature
sign
and generate the original stringsource
to be signed:- Get the signature
sign
in the raw query parameters of the request. - Remove
sign
in the query parameters and sort the remaining query parameters in alphabetical order to generate the original stringsource
to be signed.
- Get the signature
- Generate the signature: Generate the signature by encrypting
source
using the HMAC-SHA256 algorithm and the app secret obtained in the prerequisite. - Verify the signature: Compare
sign
passed through the query parameter with the signature you generate. If the two signatures are identical, the signature is considered valid. If they do not match, the signature is considered invalid.
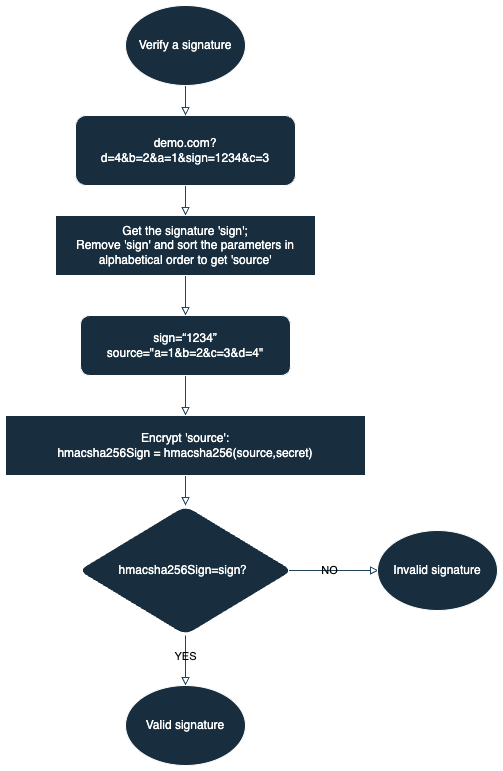
You can further verify the data's integrity by comparing the timestamp in the request query parameters with your system's timestamp when you receive the request. If the difference exceeds a specific limit (for example, SHOPLINE sets the limit to 10 minutes), consider the request invalid. This verification helps prevent the risk of intercepted requests being reused maliciously.
POST requests
Sign a request
- Generate
source
to be signed: Append the current system's millisecond-level timestamp to the request body to generate the stringsource
to be signed. - Generate a signature: Generate a signature by encrypting
source
using the HMAC-SHA256 algorithm and the app secret obtained in the prerequisite. - Add the signature to the request: Include the signature
sign
andtimestamp
in the request header.
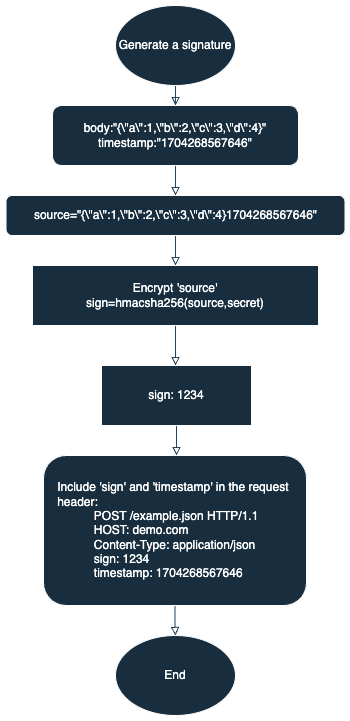
Verify a signature
- Get the signature
sign
and generate the original stringsource
to be signed:- Get the signature
sign
from the request header. - Get the timestamp and append it to the request body to generate the string
source
to be signed.
- Get the signature
- Generate the signature: Generate the signature by encrypting
source
using the HMAC-SHA256 algorithm and the app secret obtained in the prerequisite. - Verify the signature: Compare
sign
in the request header with the signature you generate. If the two signatures are identical, the signature is considered valid. If they do not match, the signature is considered invalid.
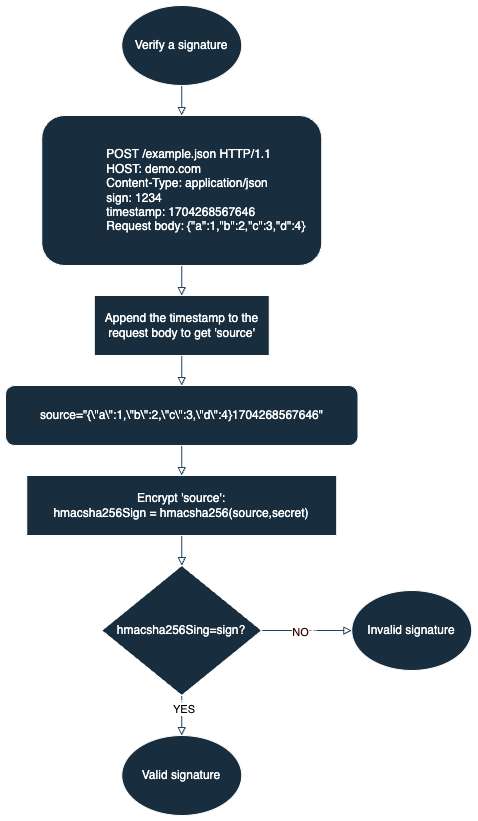
You can further verify the data's integrity by comparing the timestamp in the request header with your system's timestamp when you receive the request. If the difference exceeds a specific limit (for example, SHOPLINE sets the limit to 10 minutes), consider the request invalid. This verification helps prevent the risk of intercepted requests being reused maliciously.
Sample codes
Use the HMAC-SHA256 algorithm with your app key to sign and verify requests. The following shows some code examples of how to implement this algorithm in several common programming languages.
JAVA
package com.shopline.demo
import org.apache.commons.codec.binary.Hex;
import org.apache.commons.lang3.StringUtils;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
public class HmacDemo {
// Example usage
public static void main(String[] args) {
System.out.println(hmacSha256("The content to be signed", "Your APP Secret"));
}
/**
* hmacSha256
*
* @param source The content to be signed
* @param secret Your APP Secret
* @return
*/
public static String hmacSha256(String source, String secret) {
if (StringUtils.isNotEmpty(secret) && StringUtils.isNotEmpty(source)) {
try {
Mac sha256_HMAC = Mac.getInstance("HmacSHA256");
SecretKeySpec secret_key = new SecretKeySpec(secret.getBytes(StandardCharsets.UTF_8), "HmacSHA256");
sha256_HMAC.init(secret_key);
byte[] bytes = sha256_HMAC.doFinal(source.getBytes(StandardCharsets.UTF_8));
return new String(Hex.encodeHex(bytes));
} catch (Exception e) {
e.printStackTrace();
}
}
return null;
}
PHP
<?php
class HmacDemo {
/**
* Generate HMAC-SHA256 signature.
*
* @param string $source The content to be signed.
* @param string $secret Your APP Secret.
* @return string HMAC-SHA256 signature as a hex string or an empty string if an error occurs.
*/
public static function hmacSha256($source, $secret) {
if (!empty($secret) && !empty($source)) {
try {
return hash_hmac('sha256', $source, $secret);
} catch (Exception $e) {
error_log("Error generating HMAC-SHA256 signature: " . $e->getMessage());
return '';
}
}
return '';
}
}
// Example usage
$source = "The content to be signed";
$secret = "Your APP Secret";
echo "HMAC-SHA256 Signature: " . HmacDemo::hmacSha256($source, $secret);
?>
Python
#python 3
import hmac
import hashlib
def generate_hmac_sha256(source, secret):
if not source or not secret:
raise ValueError("Source and secret must not be empty.")
try:
# Create HMAC-SHA256 signature
signature = hmac.new(bytes(secret, 'utf-8'), msg=bytes(source, 'utf-8'), digestmod=hashlib.sha256).hexdigest()
return signature
except Exception as e:
print(f"Error generating HMAC-SHA256 signature: {e}")
return None
#Example usage
source = 'The content to be signed'
secret = 'Your APP Secret'
signature = generate_hmac_sha256(source, secret)
if signature:
print(f"HMAC-SHA256 Signature: {signature}")
else:
print("Failed to generate HMAC-SHA256 signature.")